Note
Go to the end to download the full example code.
Random Surface Points#
If only random point locations are desired, then instead of
pyransame.random_surface_dataset()
, pyransame.random_surface_points()
can be used. Compare this example to Random Surface Sampling.
import pyvista as pv
from pyvista import examples
import pyransame
antarctica = examples.download_antarctica_velocity()
The units of this mesh are in meters, which causes plotting issues over an entire continent. So the units are first converted to kilometers.
antarctica.points /= 1000.0 # convert to kilometers
sample 500 points uniformly randomly.
points = pyransame.random_surface_points(antarctica, 500)
points
array([[ 347.17330964, -1778.40600422, 0. ],
[ 637.92033519, -2045.06456725, 0. ],
[ 777.90624057, -2048.76805509, 0. ],
...,
[ 225.06692489, 2142.88450446, 0. ],
[ 501.0382241 , 2062.48241245, 0. ],
[ 614.05130864, 2086.59883196, 0. ]])
pyransame.random_surface_points()
returns a numpy.ndarray object
containing 500 point locations without any sampled data. To plot as spheres,
we first create a pyvista.PolyData object. Since we did not sample any
data from antarctica
, we do not have any scalar data, so color the
spheres red.
pl = pv.Plotter()
pl.add_mesh(antarctica, color="tan")
spheres = pv.PolyData(points).glyph(
geom=pv.Sphere(radius=50), scale=False, orient=False
)
pl.add_mesh(spheres, color="red")
pl.view_xy()
pl.show()
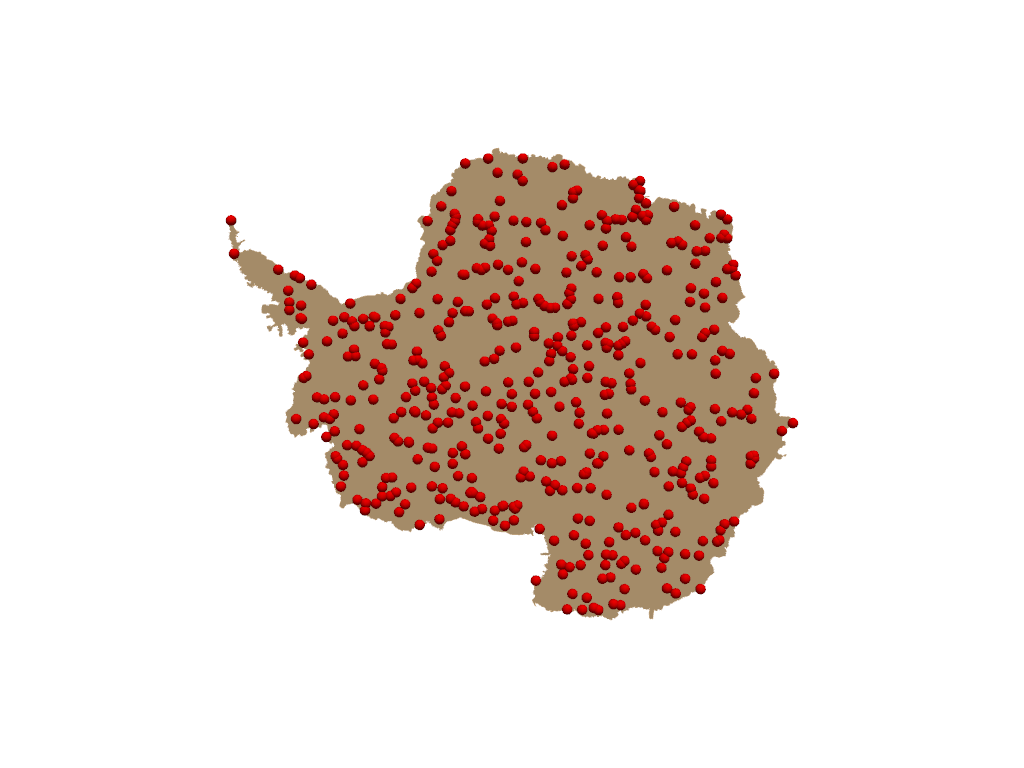
Total running time of the script: (0 minutes 8.636 seconds)